What is BankID?
BankID is a citizen identification solution used in Swedenthat allows companies, banks and government agencies to authenticate andconclude agreements with individuals over the internet. It is an electronicidentity document comparable to passport, drivers’ license and other physicalidentity documents. This practice has become a standard in Sweden and nowalmost all applications have started using bankID authentication.
Preconditions
Almost every Swedish bankissues bankIDs to their account holders. The account holders then register tothe bankID application using the given ID. Demo applications are provided todevelopers who do not have Swedish citizenship. If you are a developer, youneed to install the bankID application to your mobile or desktop to start theimplementation.
There are two types of applications;
Desktop Application
There are Mac and Windows desktop applications. Youcan install it based on your OS Preference (URL-https://install.bankid.com/). when you open theapplication it will ask for the BankID. Now we are ready to move on to the nextstep.
Mobile Application
Android: Go to https://www.bankid.com/rp/info, download and install the .apk file. When opened, the app will askfor the bankID.
iPhone: For iPhones you need to install bankIDapplication from app store.
More info: https://demo.bankid.com/
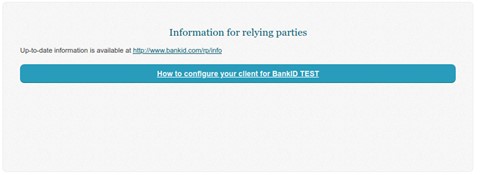
Go to https://demo.bankid.com/ and locate the area shown inthe image below.
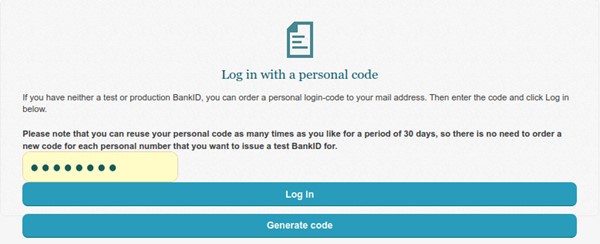
Figure 2-Create demo BankID for testing
Clickon ‘Generate code’ button and it will redirect you to the registration screen.
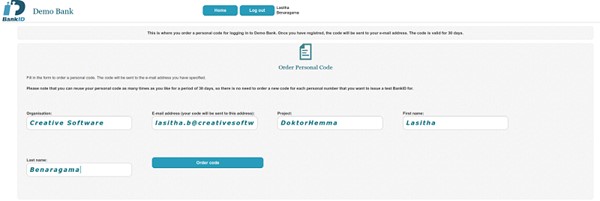
Figure 3-Bank ID request screen
Afterfilling-in the relevant details click ‘Order Code’. You will receive an emailto the provided email address.
Now you can go and graba coffee!

Figure 4-Email with code
Emailwill be in Swedish, but don’t worry, you just need the code sent in the email and as in Figure 4, you canrecognise the code - 41uyqmlo. Now copy it.
Go to https://demo.bankid.com/ and paste the code in thetext box (refer Figure 2) and click login. Locate the area shown in the imagebelow and click on the ‘Issue’ button.
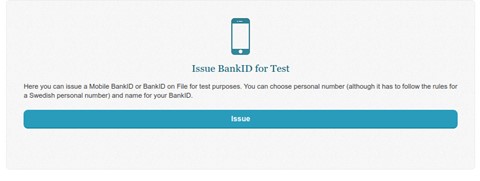
Figure 5- Issuing the Bank ID
Thenyou will be redirected to the page shown in figure 6.
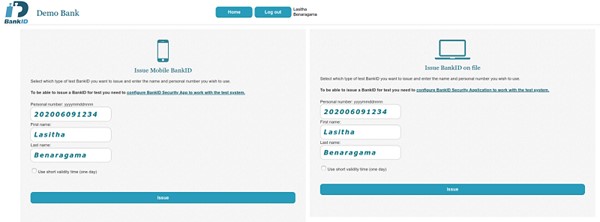
Figure6-Receiving a Bank ID with demo Social Security Number
The number format is ‘yyyymmdd’ followed by a fourdigit number. It is required to generate a Social Security Number (SSN), youcan get it by clicking here and format it according to the above.
E.g. In the URL Social Security Number is given as “Personnummer: 660509–1898” So the Preferred SSN will be 196605091898.
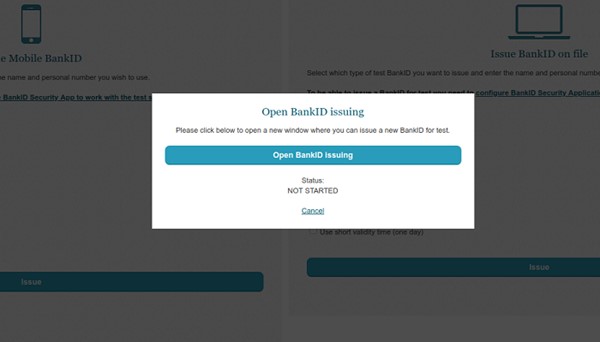
Figure 7-Step before the final stage
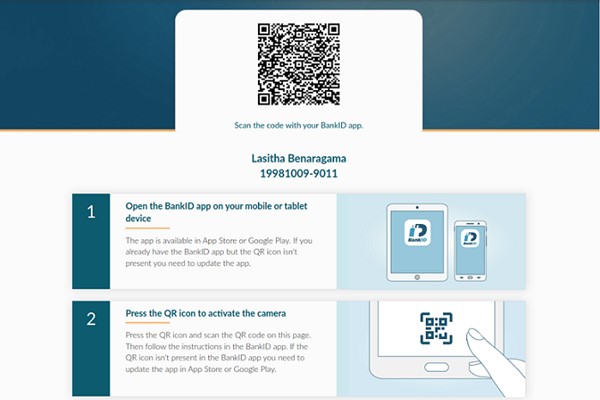
Figure 8-BankID
As shown in figure 8, open the BankID, click on QRcode button at the bottom of the screen and scan the QR Code. Then it willprompt the password screen, after entering the password and confirming it, youare DONE.
If you are using the desktop application, it willautomatically open the application and register your bank.
As for the mobile application, the process is thesame. Once you scan the QR code, password screen will be prompted. Enter andconfirm the password and you are DONE.
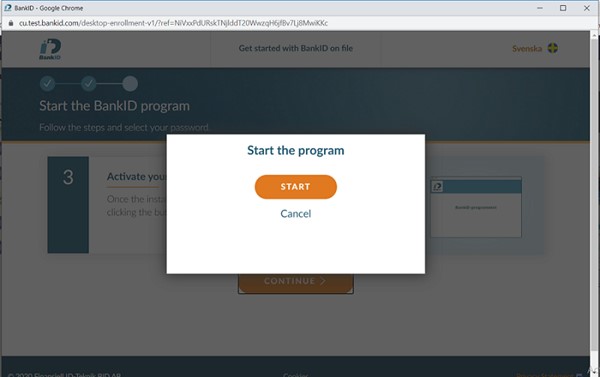
Figure 9-Seeking permission to open the Desktop Application

Figure 10-Seeking permission to open the Desktop Application
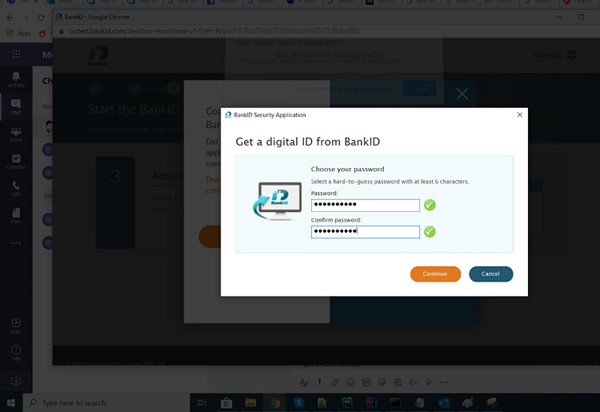
Figure 11-Password and Confirmation
Yippie...BankID is now ready. Now we can move on to the implementation part.
The Implementation process
Prerequisites
Before you begin, it is required to obtain an apikeyand authenticateServiceKey provided by the bank. The bank will also provide theapi URL to send requests.
ApiKey —wcge5b59c5n345bc41ba52ell16ef7
authenticateServiceKey —34nc567h5455hj48kl8009htj8d8e567
URL — https://client.grandid.com/
Implementation
There are three steps to complete an authentication.
· Initiate the session
· Open BankID application
· Get the user details
Initiate session
The URLs can be different from bank to bank but themechanism is the same. You basically need to call the remote URL by givingabove keys. The million dollar tip is DO NOT do it in the front end. Why? Theanswer is simple. DO NOT expose your keys. This can be achieved by creating acontroller and service in the back-end using Spring Boot to secure the APIs andthe API keys.
URL —/json1.1/FederatedLogin?apiKey={apiKey}&authenticateServiceKey={authenticateServiceKey}
Method— [POST]
@GetMapping(“/register”)
public ResponseEntity<?> createAuthenticationToken()throws Exception {
log.info(“Incoming bank registration request.”);
String autoStartToken = externalService.registerBank();
log.info(“Bank registration request executed successfully.”);
return ResponseEntity.ok(new ExternalExecutionRequest(autoStartToken));
}
Simply put, create a controller and call the remoteAPI through the back end.
public BankAuthResponse executeRemoteGetService() {
final String uri = “
Callthe remote URL using the Rest Template and the response needs to be mapped to‘BankAuthResponse’ class.
Model Class
public classBankAuthResponse {
private String sessionId;
private String autoStartToken;
public String getSessionId() {
return sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
public String getAutoStartToken() {
return autoStartToken;
}
public void setAutoStartToken(String autoStartToken) {
this.autoStartToken = autoStartToken;
}
}
Youwill get two main keys as the response.
1. SessionID — Unique key toinitiate rest of the requests
2. AutoStartToken — Token use toopen bankID Application. (Mobile/Desktop)
If you are calling the URL shown in ‘Figure 12’ withthe AutoStartToken, the browser will ask to open the bankID application. If youare using the mobile bankID application, you can create a QR code using the URLand scan it using the bankID application.
For application, you need to integrate the bank IDauthentication. You can generate a QR code in the login screen and users canscan it and login to the application you are creating.
URL to open —bankid:///?autoStartToken={autoStartToken}
Below is a sample implementation of the QR code.
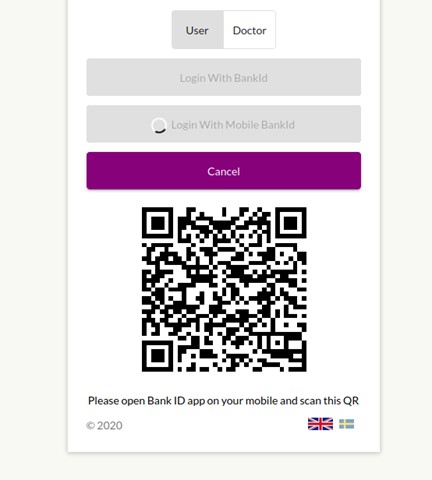
Figure 12-Sample Implementation of the QR Code
Final stage - Data Retrieval
After getting the ‘sessionID’ and ‘autoStartToken’ youneed to call another URL to get the user details. Append below URL to the APIURL and call it using the ‘restTemplate’. Refer above services.
/json1.1/GetSession?apiKey={apiKey}&authenticateServiceKey=
{authenticateServiceKey}&sessionId={sessionId}
Then you need to call data retrieval URL and after asuccessful login, it will give you a response as shown below.
{
"sessionId": "{sessionId}",
"username": "{SSN}",
"userAttributes": {
"examplekey": "examplevalue",
"...": "...",
"...": "..."
}
}
You need to call the above URL as many times asrequired until the user completes the login to BankID application. It isrecommended to call the API with a two second interval each time.
Some Tips
1. More security means more protection.You can cache the object using ‘autoStartToken’ as a key and you can hide your‘sessionID’ also.
2. There are lot of QR codegenerators in the npm sphere. Check and use the best one.
3. You can use some otherframework to call the remote API. The ‘restTemplate’ is the one provided withSpring Boot.