Spring Boot is one of the most popular Java frameworks atpresent. With convention over configuration, it makes creation of productionlevel ‘Spring applications,’ very fast and easy, therefore a lot of webapplications use this technology. This post is about validating client HTTPrequests in a Spring Boot application using HibernateValidator.
Hibernate validator contains many validators that can be usedfor validating fields. Also, we can create custom validators as required for specific checking of business logic. Let us build a simple Spring Bootapplication, and integrate the validations step by step.
1.What we will build.
Here, we will develop a simple Spring Boot application with auser registration facility. New users should be able to send HTTP post requestswith their data and register it in the system. Request validation should bedone by the server to avoid invalid data.
2.Creating a Spring Boot application.
Using your favourite IDE (IntelliJ IDEA) create a newapplication with Spring Initialiser.
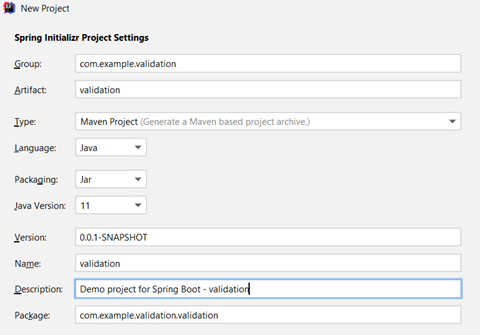
Basic POM
view rawValidating Web Requests with SpringBoot.xml hosted with ❤ by GitHub
3. DataTransfer Object (DTO)
For registration, users will send a post request. Let'srepresent it with UserRegistrationRequest.java.
view rawValidating Web Requests with Spring Boot2.java hosted with ❤ by GitHub
@NotBlank verifies that the trimmed lengthis greater than zero.
@Length gives a minimum and maximum valuefor the string.
@Email validates the correct emailformat.
The message is the output displayed if the validation fails.
In addition, there are lot more validators here.
4.The controller
Controller is the place where the client request hits first inthe server. Implement UserController with a register method as below. If any ofthe validations fail, a MethodArgumentNotValidException will be thrown.
view rawValidating Web Requests with Spring Boot3.java hosted with ❤ by GitHub
5.Generating user-friendly output.
Even though we can do the validation as above, we still don'thave a way of returning the proper error response. We need to send the list oferror messages and a suitable http error code to the client side. Let us addthe following method with @ExceptionHandler annotation into the controller forthis purpose.
view rawValidating Web Requests with Spring Boot4.java hosted with ❤ by GitHub
Here, all the MethodArgumentNotValidExceptionwill hit this method. It will return an HTTP 400 response with a list ofvalidation errors.
6.Complex validations.
Most of the time we face situations where complex validationsare needed to be done with the DTO fields. For that we need to create customvalidators. Let us introduce a new field postal code to theUserRegistrationRequest.
view rawValidating Web Requests with Spring Boot5.java hosted with ❤ by GitHub
7.Creating the validator.
Create a new Java class named PostalCodeValidator implementingConstraintValidator. Overrides initialise method with an empty body and isValidmethod with postal code validation logic which does a simple check for afive-digit number as below.
view rawValidating Web Requests with Spring Boot6.java hosted with ❤ by GitHub
Then apply the new validator in the DTO as we did under step3.
view rawValidating Web Requests with Spring Boot7.java hosted with ❤ by GitHub
8.Validating multiple fields.
Sometimes we face situations where we have to validate multiplefields. Let's add two hypothetical variables named startDate and endDate in the DTO where endDate should be afterstartDate and create a new annotation named StartEndDate.
UserRegistrationRequest.
view rawValidating Web Requests with Spring Boot8.java hosted with ❤ by GitHub
And implement StartEndDateValidator with isValid method.
view rawValidating Web Requests with Spring Boot9.java hosted with ❤ by GitHub
9.Applying the validator.
Put the new annotation in the DTO. As we are validating twofields, we have to add the annotation at the class level.
UserRegistrationRequest.
view rawValidating Web Requests with SpringBoot10.java hosted with ❤ by GitHub
10.Running the application.
Now we have created a DTO and included several validators on itsfields. Now it is time to run the application and see what happens. Let ussimply run the Spring Boot application with the IDE. Then we can send a postrequest containing a dummy UserRegistrationRequest using postman.

Because the request is valid, we do not get any error messages.Now let us try with an empty name, postal code with 6 digits and a start datewhich occurs after the end date. Now we get the list of error messages as expected.
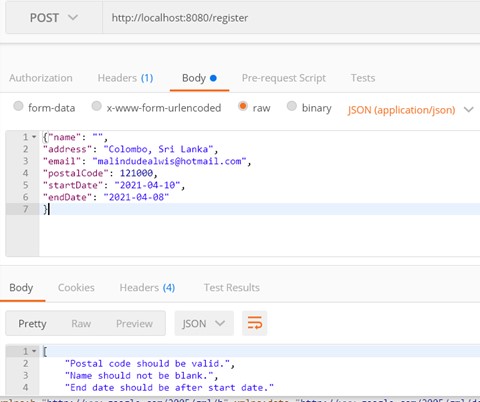
11.Conclusion.
In this way we can validate user requests using predefined /custom validators and return meaningful error messages and error codes. We can maintain clean code by minimising code duplications by reusing the validators.
You can find the complete code here.